mirror of
https://github.com/Orama-Interactive/Pixelorama.git
synced 2025-01-18 09:09:47 +00:00
Fix LightenDarken's Hue Shifting colors
- All colors move towards yellow when lighting, and purple when darkening. The logic has become more complex, so it doesn't just increase (or decrease) the hue when lighting (or darkening). This solves issues with green and blue. - Added limits to the hue when lighting and darkening, limits to the value when darkening, and to the saturation when lighting. This behavior should eventually be documented to explain how it works to the users.
This commit is contained in:
parent
397ef566db
commit
22f4796251
|
@ -44,7 +44,7 @@ Join our Discord community server where we can discuss about Pixelorama and a
|
|||
|
||||
If you like, consider helping us by sponsoring this project! It would enable us to focus more on Pixelorama, and make more projects in the future!
|
||||
|
||||
You can also support the development on patreon: [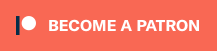](https://patreon.com/OramaInteractive)
|
||||
Toss A Coin For A New Feature: [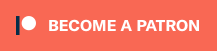](https://patreon.com/OramaInteractive)
|
||||
|
||||
## Download
|
||||
Stable versions:
|
||||
|
|
|
@ -23,6 +23,12 @@ class LightenDarkenOp extends Drawer.ColorOp:
|
|||
var sat_amount := 10.0
|
||||
var value_amount := 10.0
|
||||
|
||||
var hue_lighten_limit := 60.0 / 359.0
|
||||
var hue_darken_limit := 270.0 / 359.0
|
||||
|
||||
var sat_lighten_limit := 10.0 / 100.0
|
||||
var value_darken_limit := 10.0 / 100.0
|
||||
|
||||
|
||||
func process(_src: Color, dst: Color) -> Color:
|
||||
changed = true
|
||||
|
@ -34,18 +40,74 @@ class LightenDarkenOp extends Drawer.ColorOp:
|
|||
elif strength < 0:
|
||||
dst = dst.darkened(-strength)
|
||||
else:
|
||||
var hue_shift := hue_amount / 359.0
|
||||
var sat_shift := sat_amount / 100.0
|
||||
var value_shift := value_amount / 100.0
|
||||
|
||||
var prev_hue := dst.h
|
||||
var prev_sat := dst.s
|
||||
var prev_value := dst.v
|
||||
|
||||
# If the colors are roughly between yellow and purple,
|
||||
# reverse hue direction
|
||||
if !hue_range(dst.h):
|
||||
hue_shift = -hue_shift
|
||||
|
||||
if lighten_or_darken == LightenDarken.LIGHTEN:
|
||||
dst.h += (hue_amount / 359.0)
|
||||
dst.s -= (sat_amount / 100.0)
|
||||
dst.v += (value_amount / 100.0)
|
||||
hue_shift = hue_limit_lighten(dst.h, hue_shift)
|
||||
dst.h += hue_shift
|
||||
dst.s -= sat_shift
|
||||
dst.v += value_shift
|
||||
if dst.s < sat_lighten_limit:
|
||||
dst.v = prev_value
|
||||
dst.s = prev_sat
|
||||
dst.h = prev_hue
|
||||
|
||||
else:
|
||||
dst.h -= (hue_amount / 359.0)
|
||||
dst.s += (sat_amount / 100.0)
|
||||
dst.v -= (value_amount / 100.0)
|
||||
hue_shift = hue_limit_darken(dst.h, hue_shift)
|
||||
dst.h -= hue_shift
|
||||
dst.s += sat_shift
|
||||
dst.v -= value_shift
|
||||
if dst.v < value_darken_limit:
|
||||
dst.v = prev_value
|
||||
|
||||
return dst
|
||||
|
||||
|
||||
# Returns true if the colors are roughly between red and yellow, and purple and red
|
||||
# False when the colors are roughly between yellow and purple (includes green & blue)
|
||||
func hue_range(hue : float) -> bool:
|
||||
return hue < hue_lighten_limit or hue > hue_darken_limit
|
||||
|
||||
|
||||
func hue_limit_lighten(hue : float, hue_shift : float) -> float:
|
||||
# Colors between red-yellow and purple-red
|
||||
if hue_shift > 0:
|
||||
# Just colors between red-yellow
|
||||
if hue < hue_darken_limit:
|
||||
if hue + hue_shift >= hue_lighten_limit:
|
||||
hue_shift = 0
|
||||
|
||||
# Colors between yellow-purple
|
||||
elif hue_shift < 0 and hue + hue_shift <= hue_lighten_limit:
|
||||
hue_shift = 0
|
||||
return hue_shift
|
||||
|
||||
|
||||
func hue_limit_darken(hue : float, hue_shift : float) -> float:
|
||||
# Colors between red-yellow and purple-red
|
||||
if hue_shift > 0:
|
||||
# Just colors between purple-red
|
||||
if hue > hue_darken_limit:
|
||||
if hue + hue_shift <= hue_darken_limit:
|
||||
hue_shift = 0
|
||||
|
||||
# Colors between yellow-purple
|
||||
elif hue_shift < 0 and hue + hue_shift <= hue_darken_limit:
|
||||
hue_shift = 0
|
||||
return hue_shift
|
||||
|
||||
|
||||
func _init() -> void:
|
||||
_drawer.color_op = LightenDarkenOp.new()
|
||||
|
||||
|
|
Loading…
Reference in a new issue